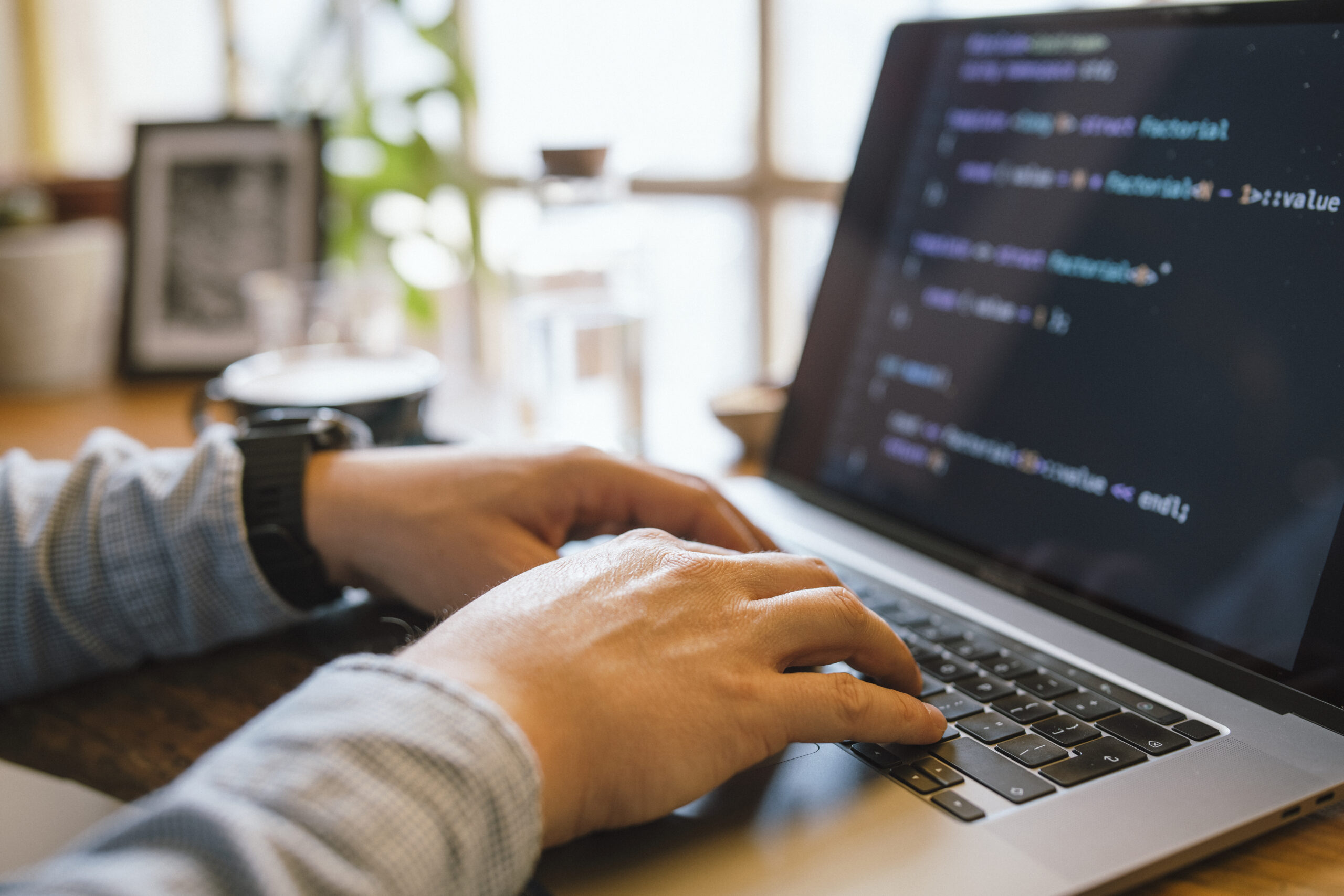
Debugging is One of the more vital — nonetheless often ignored — capabilities in the developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about understanding how and why issues go Incorrect, and Understanding to Consider methodically to resolve troubles proficiently. No matter if you are a rookie or possibly a seasoned developer, sharpening your debugging capabilities can help save hrs of stress and substantially increase your productiveness. Listed below are numerous methods to assist builders amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Resources
Among the fastest means builders can elevate their debugging expertise is by mastering the tools they use every day. Though producing code is a single A part of development, recognizing tips on how to communicate with it successfully all through execution is Similarly essential. Modern progress environments arrive equipped with highly effective debugging capabilities — but lots of builders only scratch the surface of what these instruments can do.
Choose, one example is, an Integrated Enhancement Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment permit you to established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code within the fly. When used accurately, they let you notice exactly how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-stop builders. They let you inspect the DOM, monitor network requests, perspective true-time performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and community tabs can turn discouraging UI problems into workable duties.
For backend or procedure-degree builders, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Handle about operating processes and memory administration. Learning these equipment could possibly have a steeper learning curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into comfortable with Edition Management devices like Git to understand code history, locate the exact second bugs have been launched, and isolate problematic improvements.
Finally, mastering your tools indicates going over and above default options and shortcuts — it’s about establishing an personal familiarity with your progress natural environment to make sure that when issues crop up, you’re not shed at the hours of darkness. The greater you know your tools, the greater time you can spend resolving the particular challenge in lieu of fumbling by the method.
Reproduce the challenge
The most vital — and often ignored — steps in effective debugging is reproducing the condition. Right before leaping to the code or creating guesses, builders have to have to make a steady atmosphere or scenario where by the bug reliably appears. With out reproducibility, correcting a bug will become a match of opportunity, often bringing about wasted time and fragile code changes.
The initial step in reproducing an issue is gathering just as much context as you possibly can. Talk to inquiries like: What actions brought about the issue? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or error messages? The greater depth you've, the a lot easier it gets to isolate the exact problems under which the bug happens.
When you’ve gathered sufficient facts, make an effort to recreate the condition in your local ecosystem. This could signify inputting the identical details, simulating comparable person interactions, or mimicking method states. If The problem seems intermittently, think about producing automatic exams that replicate the sting cases or condition transitions included. These checks not just enable expose the issue but in addition reduce regressions in the future.
Often, The difficulty might be setting-unique — it might take place only on selected functioning methods, browsers, or beneath unique configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a phase — it’s a frame of mind. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you happen to be now midway to correcting it. Which has a reproducible state of affairs, you can use your debugging tools more successfully, check possible fixes securely, and talk far more Plainly using your crew or end users. It turns an abstract complaint into a concrete obstacle — Which’s wherever builders thrive.
Go through and Realize the Error Messages
Error messages will often be the most beneficial clues a developer has when a little something goes Erroneous. In lieu of viewing them as aggravating interruptions, developers should master to deal with error messages as immediate communications with the technique. They usually tell you what precisely took place, in which it happened, and in some cases even why it took place — if you know how to interpret them.
Get started by looking at the concept carefully As well as in total. Many builders, especially when less than time strain, glance at the 1st line and quickly begin building assumptions. But deeper during the error stack or logs may lie the genuine root trigger. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and comprehend them initially.
Break the mistake down into components. Could it be a syntax error, a runtime exception, or possibly a logic mistake? Does it place to a specific file and line range? What module or function activated it? These questions can information your investigation and point you toward the liable code.
It’s also useful to be aware of the terminology of the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable designs, and Mastering to recognize these can dramatically increase your debugging procedure.
Some problems are imprecise or generic, As well as in These situations, it’s very important to examine the context during which the mistake happened. Check connected log entries, enter values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings either. These typically precede much larger issues and provide hints about prospective bugs.
Eventually, mistake messages are certainly not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and turn into a more efficient and confident developer.
Use Logging Wisely
Logging is Just about the most effective equipment in the developer’s debugging toolkit. When utilised proficiently, it offers true-time insights into how an software behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with knowing what to log and at what level. Popular logging concentrations include things like DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for in-depth diagnostic information and facts through progress, Data for basic occasions (like successful get started-ups), Alert for prospective problems that don’t crack check here the appliance, ERROR for actual complications, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with abnormal or irrelevant information. Too much logging can obscure vital messages and slow down your system. Deal with essential occasions, point out adjustments, input/output values, and significant choice details within your code.
Structure your log messages Obviously and consistently. Include things like context, like timestamps, ask for IDs, and function names, so it’s much easier to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what circumstances are achieved, and what branches of logic are executed—all with out halting This system. They’re Particularly precious in production environments the place stepping through code isn’t attainable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a well-imagined-out logging tactic, you are able to decrease the time it's going to take to spot concerns, get further visibility into your applications, and Enhance the Total maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not merely a technical job—it's a sort of investigation. To correctly determine and resolve bugs, developers ought to solution the process like a detective solving a mystery. This frame of mind can help stop working elaborate issues into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the signs or symptoms of the condition: mistake messages, incorrect output, or performance issues. Just like a detective surveys a crime scene, collect as much relevant information as you'll be able to without having jumping to conclusions. Use logs, check instances, and user reports to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Request your self: What might be causing this actions? Have any variations not long ago been designed on the codebase? Has this concern occurred right before underneath related conditions? The objective is to slender down opportunities and determine potential culprits.
Then, exam your theories systematically. Try and recreate the trouble in a managed surroundings. If you suspect a selected operate or component, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, request your code questions and Permit the outcomes guide you closer to the reality.
Spend shut focus to small facts. Bugs frequently disguise inside the the very least anticipated sites—just like a lacking semicolon, an off-by-a single mistake, or perhaps a race ailment. Be comprehensive and client, resisting the urge to patch the issue devoid of totally knowledge it. Short-term fixes may well hide the true trouble, only for it to resurface later on.
Last of all, preserve notes on That which you tried and acquired. Just as detectives log their investigations, documenting your debugging course of action can save time for potential challenges and assist Other folks have an understanding of your reasoning.
By pondering just like a detective, builders can sharpen their analytical abilities, technique problems methodically, and grow to be more practical at uncovering concealed problems in sophisticated devices.
Write Tests
Composing assessments is among the simplest ways to boost your debugging capabilities and Over-all development effectiveness. Assessments not simply enable capture bugs early but will also function a security net that gives you self-confidence when creating adjustments to the codebase. A very well-analyzed software is much easier to debug as it means that you can pinpoint accurately where by and when a dilemma takes place.
Get started with device assessments, which target specific features or modules. These modest, isolated exams can swiftly reveal whether or not a specific bit of logic is Doing the job as predicted. Every time a examination fails, you right away know in which to appear, significantly reducing some time expended debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear soon after previously being preset.
Following, integrate integration checks and conclusion-to-stop tests into your workflow. These assistance be sure that different parts of your software perform together effortlessly. They’re notably helpful for catching bugs that manifest in intricate methods with various elements or services interacting. If a thing breaks, your exams can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely Believe critically regarding your code. To test a attribute correctly, you require to comprehend its inputs, envisioned outputs, and edge instances. This volume of knowing The natural way prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug can be a strong starting point. Once the examination fails continually, you are able to target correcting the bug and view your examination go when the issue is settled. This technique makes certain that exactly the same bug doesn’t return Later on.
Briefly, writing exams turns debugging from a discouraging guessing activity into a structured and predictable method—supporting you capture extra bugs, quicker plus much more reliably.
Take Breaks
When debugging a tricky problem, it’s effortless to be immersed in the situation—gazing your monitor for hours, trying Answer right after Resolution. But Among the most underrated debugging applications is solely stepping absent. Having breaks allows you reset your intellect, cut down frustration, and infrequently see The difficulty from the new standpoint.
If you're far too near the code for far too very long, cognitive tiredness sets in. You would possibly start off overlooking evident problems or misreading code that you just wrote just hrs earlier. Within this state, your Mind will become a lot less successful at dilemma-fixing. A short wander, a espresso split, or perhaps switching to a different endeavor for ten–15 minutes can refresh your concentrate. Many builders report acquiring the basis of a problem when they've taken time and energy to disconnect, allowing their subconscious function during the qualifications.
Breaks also aid stop burnout, especially through more time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but will also draining. Stepping away enables you to return with renewed Electrical power plus a clearer state of mind. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
In case you’re stuck, a fantastic rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that time to maneuver close to, extend, or do some thing unrelated to code. It could really feel counterintuitive, In particular below restricted deadlines, but it in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, taking breaks is just not an indication of weakness—it’s a wise system. It gives your brain Place to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is an element of resolving it.
Discover From Every single Bug
Each individual bug you encounter is much more than simply A short lived setback—It is really an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you something beneficial in case you make the effort to replicate and review what went wrong.
Begin by asking oneself a handful of key questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with far better procedures like unit testing, code evaluations, or logging? The answers usually reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding habits moving ahead.
Documenting bugs will also be an outstanding practice. Retain a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and Anything you figured out. After some time, you’ll start to see patterns—recurring issues or popular faults—you can proactively keep away from.
In group environments, sharing what you've acquired from the bug along with your peers is usually In particular effective. Whether or not it’s via a Slack concept, a short generate-up, or a quick understanding-sharing session, encouraging Some others avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who produce ideal code, but individuals that constantly study from their errors.
In the long run, Every bug you correct provides a fresh layer towards your skill set. So future time you squash a bug, take a second to replicate—you’ll come away a smarter, far more able developer due to it.
Summary
Improving upon your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It makes you a more productive, self-assured, and able developer. The next time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.